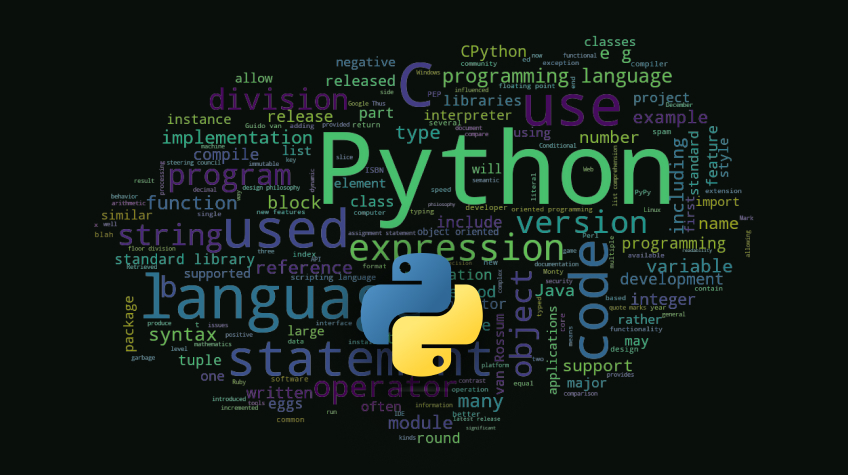
Creating a word cloud is a fun and visually appealing way to highlight the most important terms in a dataset. Whether you’re analyzing text from social media, survey responses, or any large corpus, a word cloud can provide instant insights into the most frequent words. In this article, you will go through the process of building a word cloud in Python, a versatile language that’s great for handling and visualizing data. It will cover everything from setting up your environment to customizing the appearance of your word cloud, ensuring you can tailor it to fit your specific needs.
Word clouds are more than just pretty pictures, they’re powerful tools for summarizing large amounts of text. They can quickly reveal trends and patterns that might not be immediately obvious. Python has a rich ecosystem of libraries and makes it relatively straightforward to generate these visualizations. Whether you’re an expert programmer or just starting out as a fresher, you’ll find that creating a word cloud can be both educational and enjoyable. By the end of this guide, you will have all the skills you need to create your own word clouds.
1. Install Required Libraries
First, you’ll need to install the necessary libraries. You can do this using pip:
In Bash
pip install wordcloud matplotlib
2. Import Libraries
Next, import the necessary libraries in your Python script:
In Python
from wordcloud import WordCloud
import matplotlib.pyplot as plt
3. Prepare Text Data
You need to have the text data ready. This can be any text you want to visualize, such as a document, an article, or even a string of text. For example:
In Python
text =
"""
Python is an interpreted, high-level and general-purpose programming language. Python's design philosophy emphasizes code readability with its notable use of significant whitespace. Its language constructs and object-oriented approach aim to help programmers write clear, logical code for small and large-scale projects.
"""
4. Generate Word Cloud
Use the `WordCloud` class from the `wordcloud` library to generate the word cloud. You can customize the word cloud with various parameters like `width`, `height`, `background_color`, etc.
In Python
wordcloud = WordCloud(width=800, height=400, background_color='white').generate(text)
5. Display the Word Cloud
Finally, use `matplotlib` to display the word cloud.
In Python
plt.figure(figsize=(
10
,
5
))
plt.imshow(wordcloud, interpolation=
'bilinear'
)
plt.axis(
'off'
)
# to remove the axis
plt.show()
Full Code
Here’s the complete code snippet to create a word cloud in Python:
In Python
# Import libraries
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# Sample text
text =
"""
Python is an interpreted, high-level and general-purpose programming language. Python's design philosophy emphasizes code readability with its notable use of significant whitespace. Its language constructs and object-oriented approach aim to help programmers write clear, logical code for small and large-scale projects.
"""
# Generate word cloud
wordcloud = WordCloud(width=800, height=400, background_color='white').generate(text)
# Display the word cloud
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
Additional Customization
You can further customize your word cloud by using additional parameters. Here are some examples:
✽ Change Font
Use a specific font by providing the path to the `.ttf` file.
In Python
wordcloud = WordCloud(font_path='path/to/font.ttf').generate(text)
✽ Set Maximum Words
Limit the number of words in the word cloud.
In Python
wordcloud = WordCloud(max_words=200).generate(text)
✽ Set Color Palette
Use a specific color palette for the words.
In Python
from wordcloud import ImageColorGenerator
import numpy as np
from PIL import Image
mask = np.array(Image.open("path/to/image.png"))
wordcloud = WordCloud(mask=mask, contour_width=1, contour_color='black').generate(text)
image_colors = ImageColorGenerator(mask)
plt.imshow(wordcloud.recolor(color_func=image_colors), interpolation='bilinear')
Conclusion
Creating a word cloud in Python is straightforward with the `wordcloud` library. By following the steps outlined above, you can visualize your text data effectively. Feel free to explore more customization options to make your word cloud unique and informative.