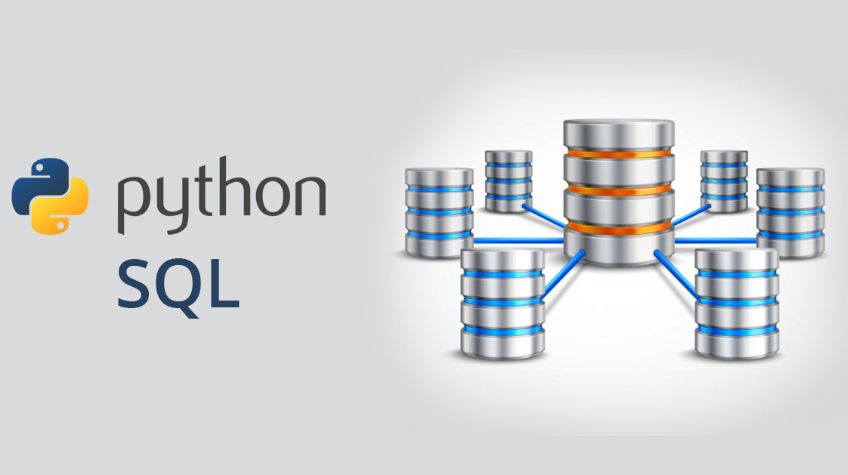
In today’s data-driven world, the ability to efficiently process and analyze large amounts of data is essential. SQL (Structured Query Language) and Python are two powerful tools widely used in data manipulation and analysis. By combining the capabilities of SQL and Python, you can leverage the strengths of both languages and streamline your workflow for efficient data processing. In this article, we will explore the process of connecting SQL with Python, enabling you to retrieve data from databases, perform complex queries, and process data seamlessly.
Table of Contents
- Why Connect SQL with Python?
- Installing Required Libraries
- Establishing Database Connection
- Retrieving Data with SQL Queries
- Performing Data Processing and Analysis in Python
- Writing Data Back to the Database
- Conclusion
1. Why Connect SQL with Python?
To start, let’s understand the advantages of connecting SQL with Python. SQL is a powerful language designed for managing relational databases. It provides efficient query processing capabilities and is widely used for data storage and retrieval. Python, on the other hand, is a versatile programming language with a rich ecosystem of libraries and frameworks for data analysis and machine learning. By combining the two, you can leverage the flexibility and ease of use of Python while harnessing the efficient data handling capabilities of SQL.
2. Installing Required Libraries
Before connecting SQL with Python, ensure that you have the necessary libraries installed. The two main libraries you will need are pyodbc and pandas. Pyodbc provides a Python DB API 2.0 interface for connecting to databases, while pandas offers powerful data manipulation and analysis tools.
To install these libraries, you can use pip, the Python package installer, by running the following commands in your terminal:
pip install pyodbc
pip install pandas
3. Establishing Database Connection
To establish a database connection in Python using pyodbc, you need to provide the necessary connection details specific to your database. These details include the database driver, server name, database name, username, and password.
For example, if you’re connecting to a Microsoft SQL Server database, you would use the following connection details:
import pyodbc
# Connection details
server_name = 'your_server_name'
database_name = 'your_database_name'
username = 'your_username'
password = 'your_password'
# Establishing connection
conn = pyodbc.connect(
f"Driver={{SQL Server}};Server={server_name};Database={database_name};UID={username};PWD={password};"
)
Make sure to replace ‘your_server_name‘, ‘your_database_name‘, ‘your_username‘, and ‘your_password‘ with the actual values specific to your database.
The pyodbc.connect() function establishes a connection to the database using the provided connection string. The connection string format may vary depending on the database you’re connecting to. Consult the documentation of your database and the appropriate pyodbc driver for the correct connection string format.
4. Retrieving Data with SQL Queries:
Once the database connection is established, you can retrieve data from the database using SQL queries. pyodbc provides a cursor object that allows you to execute SQL queries and fetch the results.
To retrieve data, you first create a cursor object using the connection:
# Creating a cursor
cursor = conn.cursor()
Next, you can execute an SQL query using the cursor’s execute() method and fetch the results using the fetchall() method:
# Executing a SQL query
query = "SELECT * FROM your_table"
cursor.execute(query)
# Fetching the results
results = cursor.fetchall()
In the above example, we execute a simple SELECT query to retrieve all rows from the table named ‘your_table‘. You can customize the query to suit your specific requirements, including specifying columns, adding conditions, or joining multiple tables.
The fetchall() method retrieves all rows returned by the query and returns them as a list of tuples, where each tuple represents a row of data.
Once you have the results, you can process and analyze the data using Python’s data manipulation and analysis libraries.
5. Performing Data Processing and Analysis in Python:
After retrieving data from the database, you can leverage Python’s powerful data processing and analysis capabilities to manipulate and analyze the data. The pandas library is particularly useful for this purpose.
To work with the retrieved data using pandas, you first need to convert it into a DataFrame, which is a two-dimensional labeled data structure. The DataFrame provides a rich set of functions and methods for manipulating and analyzing structured data.
import pandas as pd
# Converting results to a DataFrame
df = pd.DataFrame(results)
# Performing data analysis
# ... (perform your data processing and analysis tasks here)
The pd.DataFrame() function is used to create a DataFrame object from the retrieved results. The DataFrame can then be used for various data processing tasks.
With the data in a DataFrame, you can perform a wide range of operations, including:
- Data Cleaning: Handle missing values, remove duplicates, and convert data types.
- Data Transformation: Apply mathematical operations, create new columns, or reshape the data.
- Data Analysis: Perform statistical analysis, calculate aggregates, or generate summary statistics.
- Data Visualization: Create plots, charts, and graphs to visualize the data.
Additionally, Python offers a plethora of libraries and tools that can be used in conjunction with pandas for more advanced data processing and analysis tasks. For numerical computations, you can leverage the power of libraries such as NumPy. For data visualization, libraries like Matplotlib and Seaborn provide extensive plotting capabilities. For machine learning tasks, scikit-learn offers a wide range of algorithms and tools.
By combining the capabilities of SQL for data retrieval and Python for data processing and analysis, you can efficiently handle large datasets, perform complex data manipulations, and derive meaningful insights from your data.
Overall, connecting SQL with Python empowers you to seamlessly integrate database operations with the flexibility and computational power of Python, enabling you to streamline your workflow and unlock the full potential of your data analysis endeavors.
6. Writing Data Back to the Database
After processing and analyzing the data in Python, you may need to write the results back to the database. Pyodbc allows you to execute SQL statements for inserting, updating, or deleting data in the database.
# Executing an INSERT statement
insert_query = "INSERT INTO your_table (column1, column2) VALUES (?, ?)"
cursor.execute(insert_query, value1, value2)
# Committing the changes
conn.commit()
You can customize the SQL statements based on your specific requirements and execute them using the cursor object. This gives you the flexibility to write processed data back to the database.
Conclusion
In this article, we explored the process of connecting SQL with Python to streamline your data processing workflow. By combining the power of SQL for data retrieval and Python for data manipulation and analysis, you can efficiently process and analyze large datasets. Establishing a connection, retrieving data with SQL queries, performing data processing and analysis in Python, and writing data back to the database are essential steps in this workflow. With the knowledge gained from this article, you can leverage the strengths of both SQL and Python to unlock the full potential of your data processing tasks.
Connecting SQL with Python provides a powerful combination that can significantly enhance your ability to handle and analyze data efficiently. By leveraging the strengths of both languages, you can streamline your workflow and gain valuable insights from your data. Whether you are working with large datasets or performing complex data manipulations, connecting SQL with Python is a valuable skill to have in your toolkit. Start integrating SQL and Python in your data processing workflow and unleash the true potential of your data analysis endeavors.